Easily build powerful user management, authentication, and authorization into your web and mobile applications. Download this Forrester report on the new landscape of Customer Identity and Access Management, brought to you in partnership with Stormpath.
Recently, the Developer Preview of Angular 2 was released. This has some essential changes compared to Angular 1. Angular 2 is written entirely in Typescript and meets the ECMAScript 6 specification. Although one can’t build an entire application with it, because some specifications have not been implemented yet or are still subject to change, the new way of thinking already becomes clear.
Within the Angular community, there is not always a consensus, which already led to various contributors leaving the team. Angular 2 will be a big change for developers compared to 1.x. Component-based development and object orientation are much easier, which I'll explain later. Other functionalities such as scopes and controllers are canceled completely.
Component-Based
Angular 2 is entirely component based. Controllers and $scope are no longer used. They have been replaced by components and directives. Components are directives with a template. The following code snippet, Listing 1, shows how a component is built. This component is in HTML called with the <angularComponent> tag, the name of the selector within the HTML.
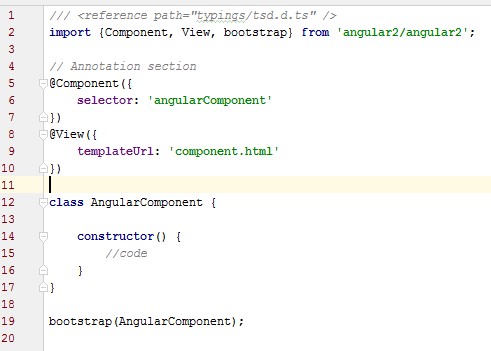
All of the components that are used must be made known via bootstrap. They also have to be imported on the page.
Directives
The specification for directives is considerably simplified, although they are still subject to change. With the @Directive annotation, a directive can be declared. This is shown in Listing 2. This is a limited set of possibilities. In the block 'host listeners', standard functions can be linked to their own implementations.
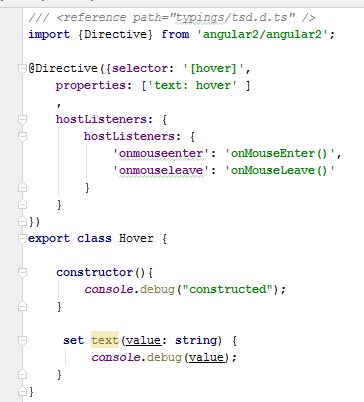
Listing 2
Directives can also be used in components, this applies both to its own directives as predefined directives. For example, the "NgFor" and "hover" directive in a component can be used. These will be imported initially and then made known within the @View.
Dependency Injection
Because of the improved dependency injection model in Angular2 there are more opportunities for component / object-based work. The dependency injection consists of 3 parts. The Injector, which contains the APIs to inject the dependencies and make dependency injection available. Bindings make it possible for dependencies to be named. Finally the actual dependencies of the object are generated so they can be injected.
At the moment, an object is passed into the constructor of the component, this only needs to be passed through the injector view, as shown in Listing 3. The objects that are enclosed in the square brackets can then be used to inject.
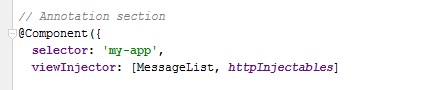
Listing 3
Some extra annotations have been added which can enhance the possibilities of dependency injection, for example the @InjectPromise annotation. With this annotation asynchronicity can be achieved, and the object is only injected as it actually has been created. At this moment a specific Promise object will be injected. With the @optional annotation, an optional value or library can be injected.
An example of dependency injection is the following declaration:

A special application of dependency injection is HTTP. Through the use of the httpInjectables, which exist in the angular/http package, a HTTP object can be injected. On this object, REST operations can be performed. For the example, a public test server with a REST response has been used. It can be called like this:
http.get('http://jsonplaceholder.typicode.com/posts/1')
What is TypeScript and What are the Benefits of ECMAScript 6
EcmaScript 6 has classes, a typical example of a class is (Listing 4):
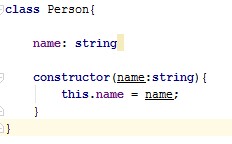
Listing 4
In previous versions of ECMAScript, everything was still defined by a prototype. Now classes are defined and it makes it almost as readable as Java code. ECMAScript 6 also has full inheritance. With super (parameters) to the constructor of the parent is called. Furthermore, static variables can be declared.
TypeScript is an extension of ECMAScript, in fact:
TypeScript = ES6 + Types + Annotations
TypeScript is actually from Microsoft, which means the new Angular is also likely to be popular for .NET developers. TypeScript is a form of JavaScript which knows types and classes and can be compiled to JavaScript. It is open source. TypeScript includes many aspects of object orientation such as inheritance and interfaces. It also has generics and lambdas.
Because Angular2 uses TypeScript, the functionality of TypeScript itself and its libraries can be used. Angular is just a framework which couples different features. Other libraries can easily be used. For example, you can use the MongoDB interface because it already has a connector in TypeScript.
WebSockets is another feature that is not directly something from Angular2, but it can easily be implemented and support has been added to the specification. We can define a WebSocket like this:
On the socket we can receive messages by defining an onMessage() function. We can also emit changes. If we build a WebSocket server we can get real-time changes.
TypeScript will not be used in the browser. The program code is compiled to JavaScript. This can be achieved with “Traceur”. Because the JavaScript ECMAScript 6 specification has also needed an additional plug-in to be used because the browsers are not yet suitable for this purpose.
Generics
TypeScript has generics which can be used in the frontend. As an example uses a Male and Female class which are both derived from Person (Listing 5). Both can prepare Food, however, the result is different. One only seems to be able to prepare raw food and the other can make delicious food.
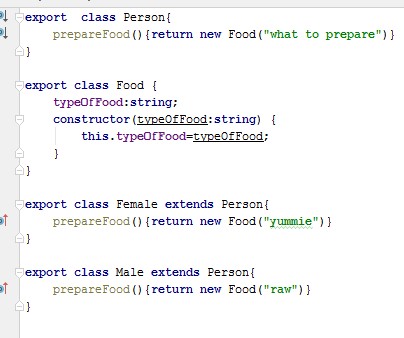
Listing 5
In Angular, every class or directive that is used in a component that must first be imported in the component before it can actually be used. In listing 6, the NgFor directive has been imported to use all classes for the generics.
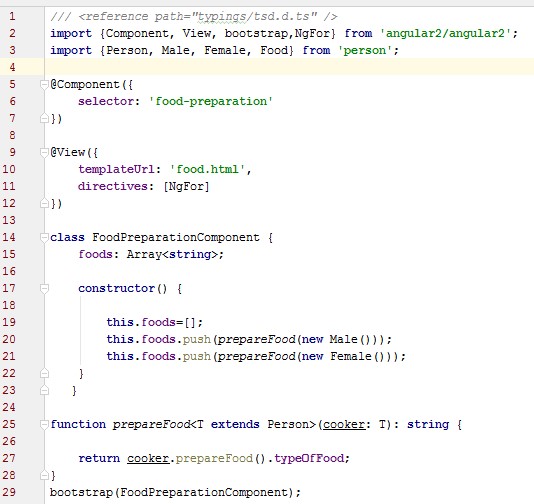
Listing 6
The "foods" list is now displayable with Angular, the following piece of HTML has been used:
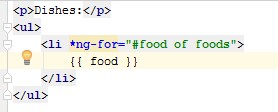
Listing 7
The browser then displays a list. This example is relatively simple, later when we discus Forms we will see more advanced options will be possible.
Lambdas with TypeScript
In TypeScript, lambdas are available. The following piece of code makes of every string in messageStrings a new message with this string as content. The string is than pushed in the messages list.
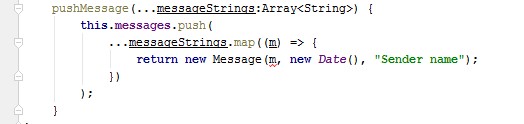
Listing 8
Another application of lambdas is the HTTP GET. Http get supplies a not-directly-usable object, but a RxJS subject. With help of the toRx() method, we can transform it to an Observable. After this we can subscribe to this observable by using the .subscribe. Every change can now be mapped.

Forms and Validations
Forms and validations are an important aspect of frontend development. Within Angular 2 the Form Builder and Control Group are defined. The Control Group consists of a list of Control. Listing 9 shows how two fields are added to the Control Group. Each addition is a Control and as could be written as new Control();
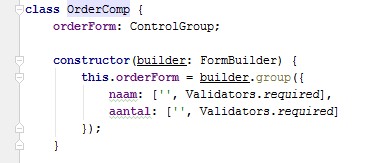
Listing 9
The empty field is the initial value, but this can of course also use a value from the database. Next, this can be called in the HTML like this.
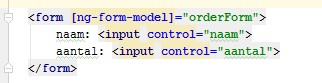
Listing 10
Now we can access the errors of each control like this, "<<this.orderForm.controls.naam.errors >>”.
We can also define ControlGroups within ControlGroups, this allows validation of fields on Form level, on validation is on Form level as being a control group, the next nesting contains the actual controls. This validations can then be invoked with “<<this.orderForm.errors>>".
Each Control or validation can have its own implementation. This makes it possible to build your own library with personal functions and controls. Reuse will be easy to achieve. Combined with generics and inheritance it is possible to define a date which uses a rule with generics. Now with inheritance and different types of rules it’s possible to define a pallet of controls. For example, the generic rule is the date is the right size, the line added with generics, the date must be at least 18 years in the past.
Also the Forms and Controls still undergo changes and it is expected that more standard operations will be included.
More Possible
At this moment, not all specifications are fully implemented and are still changing. The direction is very clear, but at this moment, a minor release could cause a failure in your code.
It’s a big step forward that the frontend is now completely made of components and directives and can use all the features from other JavaScript libraries. Is it easy to communicate with the database and make use of WebSockets. It has also become a better way to create directives and components that are very easy to integrate with other frameworks. It is no longer a typical JavaScript framework, it is compiled to JavaScript, but in fact, every language may be used if it can be compiled to JavaScript.
The combination of Angular2 with TypeScript provides a big opportunity in object oriented programming in the frontend. The declarative nature makes it much clearer.
All of this looks very promising and probably will change the way we develop frontends. We probably need to wait until all frontend implementations are finished.
ReplyDeleteWonderful blog & good post.Its really helpful for me, awaiting for more new post. Keep Blogging!
Hire Angular Developer in India